Elements
Photon Elements are a set of prebuilt UI-components that can be used to add prescribing functionality into any web-based clinical tool.
Elements are built using WebComponent technology and can be used in any frontend app - regardless of framework. Elements do not use outdated iframe
technology!
Elements automatically handle authentication and ensure that only authorized prescribers can send prescriptions. The UI is designed to be responsive across various screen sizes.
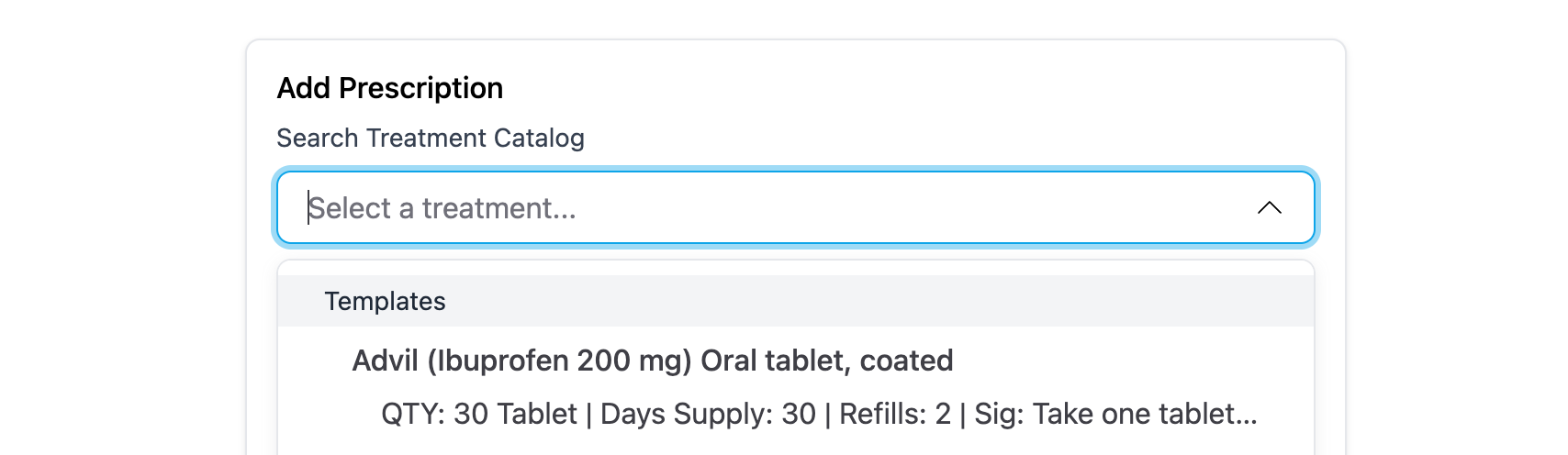
This UI can be embedded directly in your clinical app.
<photon-client
id="YOUR_CLIENT_ID"
org="YOUR_ORG_ID"
>
<photon-prescribe-workflow/>
</photon-client>
Get Started
First install our elements package from NPM:
npm i @photonhealth/elements
If using a framework like React, you'll need to dynamically import the package. This will register the custom elements with the browser's CustomElementRegistry
.
import("@photonhealth/elements")
See this repository for a demonstration of using the elements within a React app.
Client Element
Next, add the photon-client
element toward the top level of your application.
<photon-client
id="your_client_id"
org="your_org_id"
redirect-uri="https://localhost:3000"
dev-mode="true"
>
<!-- nested photon elements -->
</photon-client>
Property | Description |
---|---|
id | The public ID registered to your application (with whitelisted URLs) |
org | The Photon organization ID associated with your account |
auto-login | Optional, defaults to "false". Set this to auto-login="true" to trigger automatically login on page load if the user is unauthenticated |
redirect-uri | Optional. Specifies where to redirect the user after a successful login. This cannot include a pathname, so for example it should look like https://example.com rather than https://example.com/user/1234/prescribe . You can however incorporate a strategy that handles a query parameter such as https://example.com?redirect=user%2F1234%2Fprescribe .When working locally, set the port to 3000 for authentication to work. |
redirect-path | Optional. Helper that reroutes to a pathname after logging in returns the user to the redirect URI. An example redirect path is user/1234/prescribe . This will only work if the photon-client element exists at the redirect-uri base path. |
dev-mode | Optional. When working locally set this to "true" in order to point our service at our staging service neutron rather than our production services at photon |
These values can be found in the settings page of the Photon App, or in the Sandbox App. All photon-
elements must be contained within the photon-client
to function correctly.
If you're unable to put the photon-client
at the root of your domain, then the property redirect-path
will not work. However you can pass a query parameter to the redirect-uri
property such as https://localhost:3000?redirect=user/1234
and then handle the redirect with your own application logic.
Check out our example repo that shows how to handle the authentication redirect. The main
branch shows how to use a query string to redirect and the root
branch shows how to use the photon-client
at root with the redirect-path
.
Prescribe Element
The photon-prescribe-workflow
can be added anywhere within the photon-client
and renders a basic prescribe workflow. It accepts the following props:
Property | Description |
---|---|
patient-id | ID of the patient receiving the prescription |
template-ids | Templates that should be pre-selected |
template-overrides | When adding template-ids this attribute allows for overriding specific fields on the template. The overrides are a mapping where the keys are template IDs and the values are the override specifications.<photon-prescribe-workflow template-ids="tmp_01H5JB37PPK9F64RE3QQ52WD7M,tmp_01GQJDV39GPEJ03BATZV1X0SRJ" template-overrides='{"tmp_01H5JB37PPK9F64RE3QQ52WD7M": {"instructions": "these instructions are overriding the previous instructions", "fillsAllowed": 200}}' /> |
additional-notes | Setting this parameter will append the string to each draft prescription's notes. Providers can still edit these notes. |
prescription-ids | Prescriptions that should be pre-selected |
enable-order | Boolean that enables the creation of an order at the same time as a prescription. When this flag is set with nothing else, a pharmacy selection card will default to a "Local Pickup" option. |
enable-send-to-patient | Prerequisite enable-order set to true .Boolean that allows prescribers to send patients the option to set their own pharmacy. |
enable-local-pickup | Prerequisite enable-order set to true .Boolean that allows prescribers to set the patient's pharmacy for them. |
enable-coverage-check | Boolean that allows a patient's insurance coverage to be checked for prescriptions added in the prescribe workflow. For a selected or nearby pharmacy, the prescriber will be shown a medication covered status, therapeutic and pharmacy alternatives, Prior Authorization warnings, and an estimated copay. Requires patient benefit data to be on file with Photon. Please reach out to us for assisrtance configuring this. |
mail-order-ids | Prerequisite enable-order set to true .Comma separated list of mail order pharmacy ids. |
pharmacy-id | Prerequisite enable-order set to true .Sets a preselected pharmacy. |
hide-templates | Optional boolean, defaults to false . Setting this flag to true will remove the ability for providers to save personal templates. |
hide-submit | Optional boolean, defaults to false . Setting this flag to true will remove either the "Save Prescriptions" or "Send Order" button depending on your configuration. |
hide-patient-card | Optional boolean, defaults to false . Setting this flag to true will hide the patient card from the prescribe flow. |
address | Optional, setting address will override the prescriber's ability to manually set the the patient's address when enable-order is set to true . The address object to be passed takes this shape:{ city: string; postalCode: string; state: string; street1: string; street2?: string; country?: string; } Please note that web components accept attributes as strings. Therefore, when passing an object as a prop, you must stringify it first. Here's an example of how to pass the address object to a web component: address={JSON.stringify(address)} |
external-order-id | Optional ID that can be associated with the order being created. |
enable-med-history | Optional Boolean, enables a card in the prescribe flow that shows the selected patient's recent prescriptions. |
enable-med-history-links | Optional Boolean, enables a link on the medication row that directs to Photon's Prescriber Portal. |
enable-med-history-refill-button | Optional Boolean, enables a button to add an existing prescription to the order. |
enable-combine-and-duplicate | Optional Boolean, enables a recent orders card that shows prescriptions recently sent. If they seem to be duplicate, we'll notify the prescriber. Also, if the order hasn't been sent to a pharmacy yet we'll attempt to combine the orders into a single order to make for better patient communication. |
weight | The weight of the patient, number. |
weight-unit | The weight unit, either lbs or kg . |
Here's an example of a Photon Element that enables orders to a preselected pharmacy:
<photon-prescribe-workflow
patient-id="pat_9876zyxw"
enable-order="true"
pharmacy-id="phr_1234abcd"
/>
Alternatively, here's how you would enable an prescribe element with all of the pharmacy selection options:
<photon-prescribe-workflow
patient-id="pat_01GQ0XFBHSH3YXN936A2D2SD7Y"
enable-order="true"
enable-send-to-patient="true"
enable-local-pickup="true"
mail-order-ids="phr_01GA9HPVBVJ0E65P819FD881N0"
/>
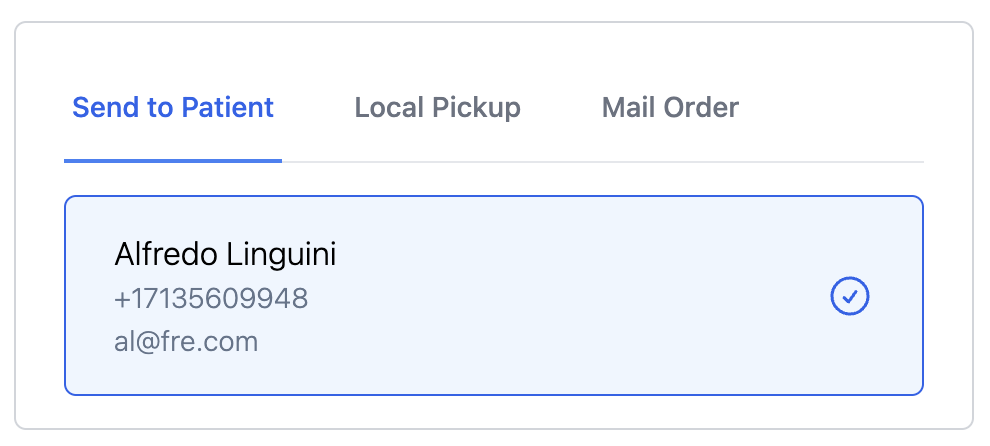
The above code will enable a similar Pharmacy Select card.
Events
Event payloads will be on detail
.
document.addEventListener('photon-order-created', (e) => {
console.log('Order Info: ', e.detail.order);
});
Event Name | Description | Detail |
---|---|---|
photon-prescriptions-created | Triggered when prescriptions have been successfully created. | { prescriptions: Prescription[] } |
photon-order-created | If the enable-order flag is set and the prescriber selects to "Send Order", this will trigger when prescriptions and an order are successfully created. | { order: Order } |
photon-prescriptions-error | Triggered when there are errors creating prescriptions. | { errors: GraphQLError[] } |
photon-order-error | Triggered when there are errors creating the order. | { errors: GraphQLError[] } |
photon-draft-prescription-created | Triggered when a draft prescription is added to the "Pending Order" card. | { draft } |
photon-draft-prescription-deleted | Triggered when a draft prescription has been removed from the pending orders section. Returns the deleted prescription. | { prescription } |
photon-order-combined | Triggered when a draft prescription has been combined into an existing order. | { orderId } |
Medical History Component
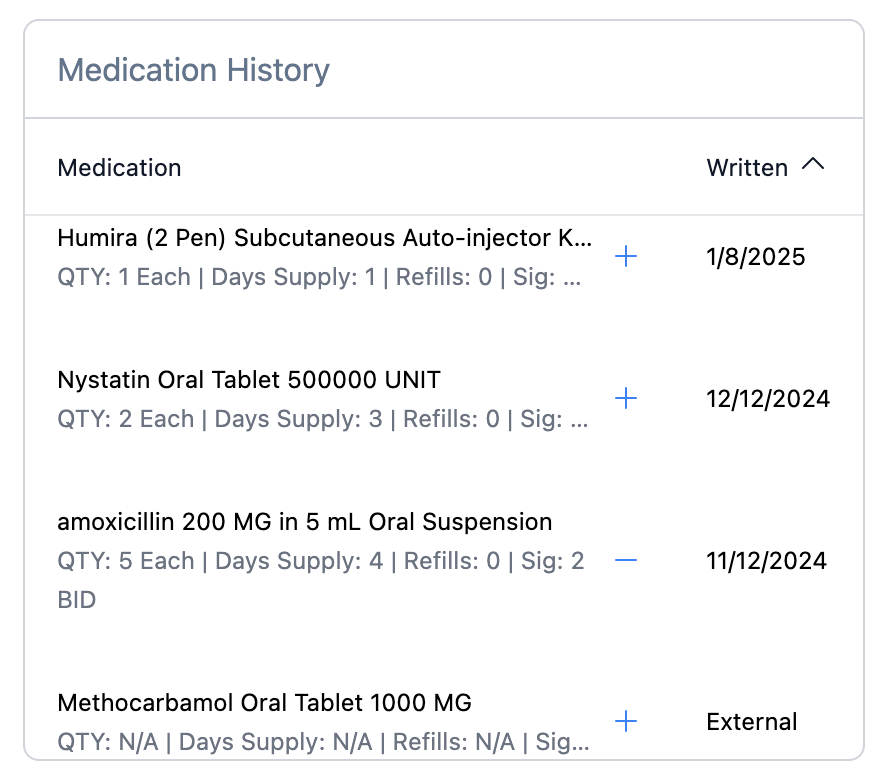
The photon-med-history
can be added anywhere within the photon-client
and renders a list of the patients most recent prescriptions. It accepts the following props:
Property | Description |
---|---|
patient-id | Accepts a patient's Photon ID or their external ID |
<photon-med-history patient-id="123abc"></photon-med-history>
Framework Support
Below you'll find example integrations for React, Angular, and Vue.
import("@photonhealth/elements").catch(() => {});
import { createRef, useEffect, useState } from "react";
function App() {
const photonPrescribeRef = createRef();
useEffect(() => {
const log = () => {
console.log("treatment prescribed!");
}
if (photonPrescribeRef.current) {
photonPrescribeRef.current.addEventListener("photon-prescribe-success", log);
const removal = () => photonPrescribeRef.current.removeEventListener("photon-prescribe-success", log);
return () => removal()
}
}, [])
return (
<div>
<photon-client
id="CLIENT_ID"
org="ORGANIZATION_ID"
>
<photon-prescribe-workflow ref={photonPrescribeRef} />
</photon-client>
</div>
);
}
export default App;
import { Component } from '@angular/core';
import('@photonhealth/webcomponents').catch(() => {});
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'my-app';
public log() {
console.log("treatment prescribed!");
}
}
...
<div>
<photon-client
id="CLIENT_ID"
org="ORGANIZATION_ID"
>
<photon-prescribe-workflow (photon-prescribe-success)="log()"></photon-prescribe-workflow>
</photon-client>
</div>
<script setup lang="ts">
import("@photonhealth/webcomponents").catch((err) => {});
const log = () => {
console.log("treatment prescribed!");
}
</script>
<template>
<div>
<photon-client
id="CLIENT_ID"
org="ORGANIZATION_ID"
>
<photon-prescribe-workflow v-on:photon-prescribe-success="log()" />
</photon-client>
</div>
</template>
Updated about 2 months ago